Basics of HTML and CSS
HTML is the language that powers websites. You use HTML to structure the content that shows up on the webpage. CSS is the language that handles styling of the webpage and content layout. We will be making a basic todo list in this tutorial, so lets get started! Please follow along by writing the code yourself and using your browser to open the file. Make sure to refresh the webpage when you’ve saved your code.
Table of Contents
A Basic HTML Page
Open up your favorite code editor (I like to use Visual Studio Code). Make a new file and call it something.html
. All html files end in either .html
or .htm
. First we put <!DOCTYPE html>
at the top of the file. This will tell the browser that we are using the latest version of HTML. Next let’s put a html tag in the document.
1 <!DOCTYPE html>
2 <html>
3 Stuff inside of the html tag
4 </html>
As you can see, a html element has an opening (<html>
) and closing (</html>
) tag. Inside of the opening and closing tag are either other tags or content. Usually tags inside of other tags are indented by a tab or 4 spaces to allow for easier code reading.
The head element stores information that isn’t shown to the webpage reader, but has information for search engines and links to styling resources and the title of the webpage. Let’s add a title to our html file:
1 <!DOCTYPE html>
2 <html>
3 <head>
4 <title>This is the title of my website!</title>
5 </head>
6 </html>
Like a letter webpages have a head and a body. The body is where the content that you see on the webpage is stored.
1 <!DOCTYPE html>
2 <html>
3 <head>
4 <title>This is the title of my website!</title>
5 </head>
6 <body>
7 Hello, my amazing readers! You are fantastic!
8 </body>
9 </html>
A Basic Todo List
Doesn’t everyone always have too much on their plate? Let’s make a simple todo list to lessen our stresses. In html, the ul
tag creates a bullet list and the ol
tag creates a numbered list. Inside of those tags you use li
tags which contain the text you want to put on your list.
1 <!DOCTYPE html>
2 <html>
3 <head>
4 <title>This is the title of my website!</title>
5 </head>
6 <body>
7 Why do I have so much to do...
8 <ul>
9 <li>Write an article</li>
10 <li>Write another article</li>
11 <li>Write 2 more articles</li>
12 <li>Read a few articles</li>
13 <li>Write 3 more articles</li>
14 </ul>
15 The work never ends. :(
16 </body>
17 </html>
A Prettier Todo List
Honestly, my todo list is too plain to look at. Let’s use some CSS to stylize it and make it prettier. CSS is fairly simple. There are selectors which tell the browser what html elements to apply the styles to and properties which can change certain styles of our elements. We will go over some selectors and properties in a bit.
1 li {
2 color: red;
3 }
4 .this-is-a-class-selector {
5 color: blue;
6 }
7 #this-is-an-id-selector {
8 color: green;
9 }
On line 1, we can see the selector is the li
html element. This means that for every li
element in our html the color will be red. On line 4, the selector is for CSS classes.
A html element like this <li class="this-is-a-class-selector another-class">Hi</li>
will have the styles from this-is-a-class-selector
and another-class
applied to it. But wait, we already changed a color for all li
elements! What happens when we change the color again with a class? I’m glad you asked, dear reader. The later things in your CSS take precedence over the things before it. So li
elements that don’t have a class will be red, but li
elements with a class will be blue because that style is written later in the CSS.
On line 7, that is an id selector. Only one of those can be applied per element unlike classes. This is how they are applied: <li id="this-is-an-id-selector">Hello again</li>
. Usually you’ll see most web developers using classes everywhere even if there is only one selector needed because it’s easier to add more styles with different classes later.
Phew, that was a lot of CSS information. Let’s actually color our todo list now. Inside the head tag we’ll create a style tag where we put our CSS code.
1 <!DOCTYPE html>
2 <html>
3 <head>
4 <title>This is the title of my website!</title>
5 <style>
6 li {
7 color: red;
8 }
9 .this-is-a-class-selector {
10 color: blue;
11 }
12 #this-is-an-id-selector {
13 color: green;
14 }
15 </style>
16 </head>
17 <body>
18 Why do I have so much to do...
19 <ul>
20 <li class="this-is-a-class-selector">Write an article</li>
21 <li id="this-is-an-id-selector">Write another article</li>
22 <li>Write 2 more articles</li>
23 <li>Read a few articles</li>
24 <li>Write 3 more articles</li>
25 </ul>
26 The work never ends. :(
27 </body>
28 </html>
You did it! You now have a colorized todo list. :) For more cool CSS styles, just search the web for things that you want to do. e.g. css font size. Here are a few interesting ones to get you started:
- background-color
- font-family
- font-size
Check out other posts in this series
Join me in learning about how computers work
Just enter your email into the box below. You'll also receive my free 12 page guide, Getting Started with Operating System Development, straight to your inbox just for subscribing.
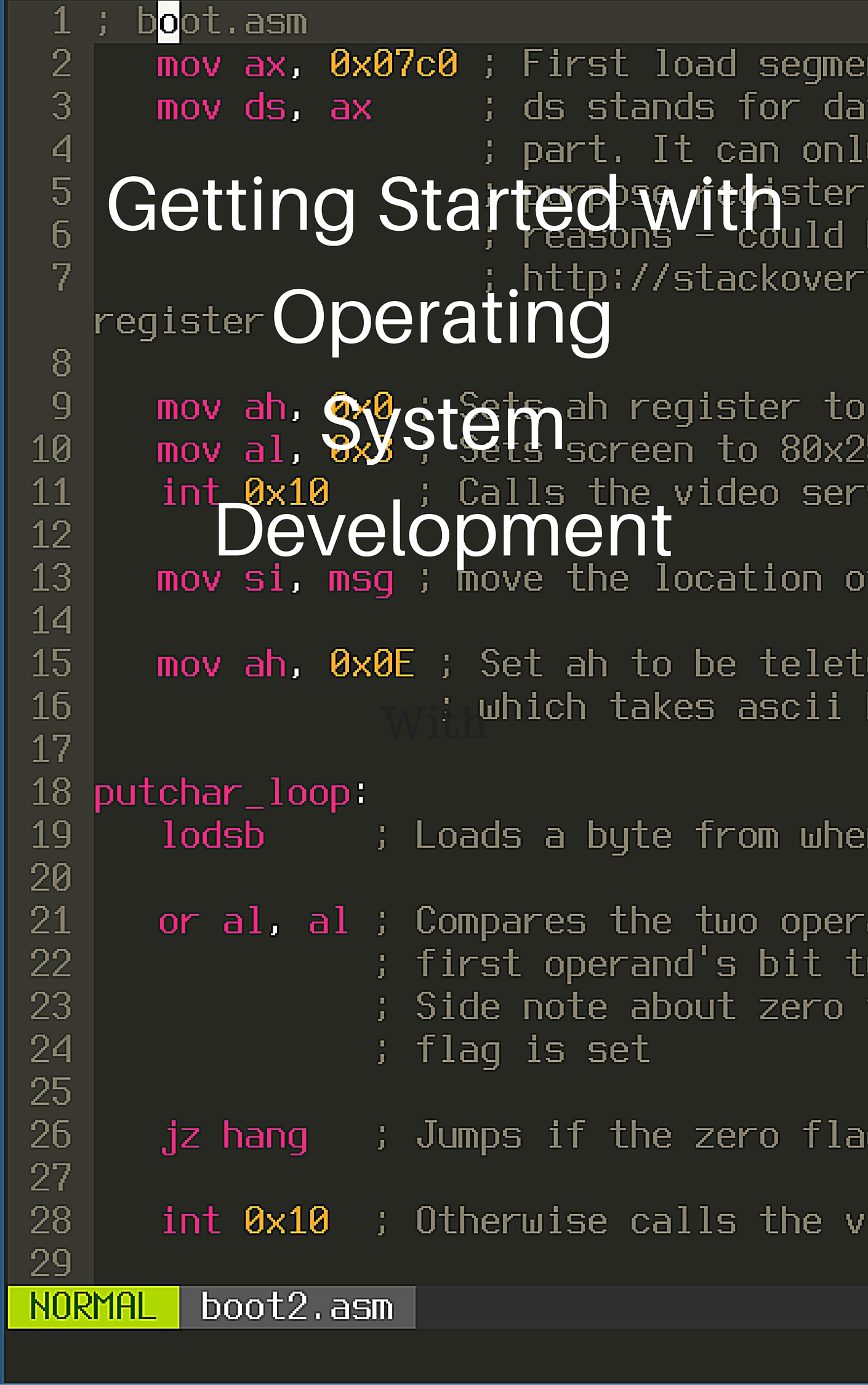